Python allows you to run shell commands which you can use in your automation tasks and manage to start other serverside programs . Some of the useful python modules to execute shell commands are :
os.system(), os.popen() and subprocess.Popen
You can immedeatley execute a shell command with the os.system command
import os
os.system('shell command')
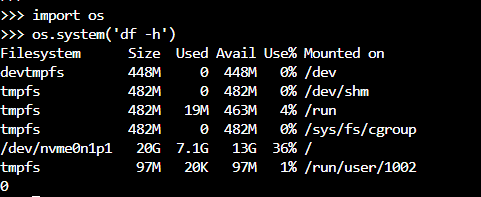
os.popen module opens a pipe from command prompt . This pipe helps the command to send its output to another command . The output will be an open file and can be accessible from other programs .
Read(r) ::pipe = Popen('cmd', shell=True,bufsize=bufsize,stdout=PIPE).stdout
Write(w)::pipe = Popen('cmd', shell=True, bufsize=bufsize, stdin=PIPE).stdin
import os
p = os.popen('df -h')
print(p.read())
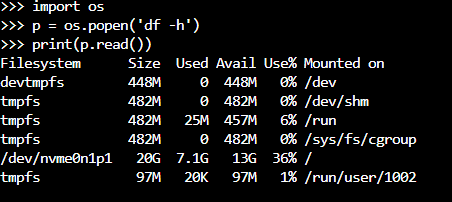
It is better to assign the shell command to a variable and then use a seperate variable to interact with it . This will keep it neat as below:
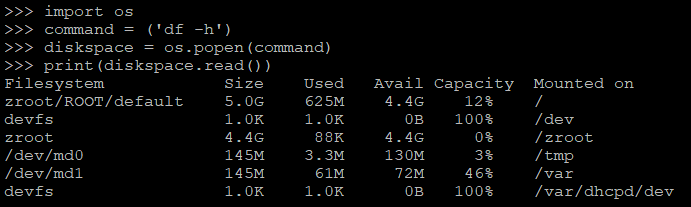
Pythons subprocess modules replaces many features of the os modules. This module has the Popen class . This method allows the execution of the commands as child process. Some of the important subprocess.Popen methods are :
Popen.poll() Checks if the child process has terminated.
Popen.wait() Wait for the child process to terminate.
Popen.communicate() Allows to interact with the process.
Popen.send_signal() Sends a signal to the child process.
Popen.terminate() Stops the child process.
Popen.kill() Kills a child process.
The available parameters for subpocess.Popen are :
subprocess.Popen(args, bufsize=0, executable=None, stdin=None, stdout=None, stderr=None, preexec_fn=None, close_fds=False, shell=False, cwd=None, env=None, universal_newlines=False, startupinfo=None, creationflags=0)
For eg : if you want to execute the command is shell , you can use the parameter shell=True
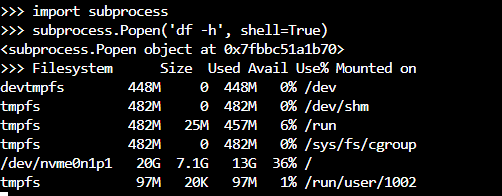
You might have noticed the object 0x7f.. in the last command , its better to assign the command to variable and execute in that way to keep it clean as below :
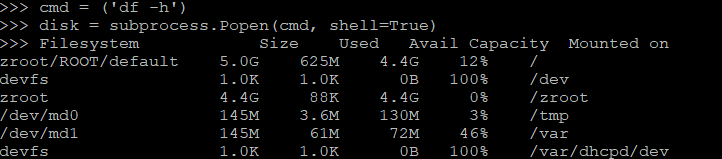
If you want to append the output of a command to a log file , check the link below :
The poll() method returns the exit code if a command has finished running, or None if it’s still executing. For example:
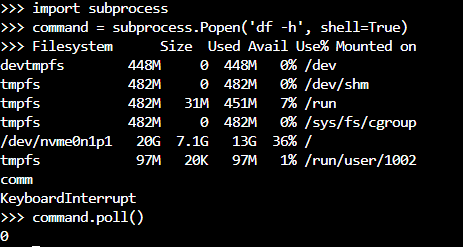
the communicate() is another important method to communicate with the input and output of a command. You can use the ouput of one shell command to another one using this method .

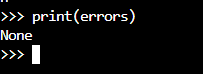
If you want the ouput to be properly formatted just use the decode function before printing the output:
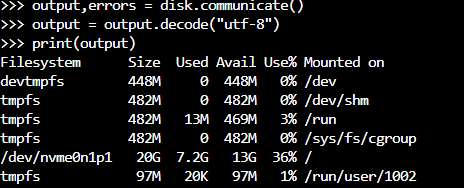
Leave a Reply